qt8gt0bxhw|20009F4EEE83|RyanMain|subtext_Content|Text|0xfbffc50000000000b000000001000200
At times I'll build a suite of related, but separate applications. Even though each application is a separate executable, I like to be able to integrate the applications so they can work together. Sending messages between your applications is a great way to do just that. I build this kind of thing into my apps often, but really think nothing of it since the code is hidden in a base form class. I was asked by someone how to communicate with other apps so I decided to blog a sample to answer the question (which is where most of my posts come from it seems).
There are two parts to communicating via messages. First, you need to be able to listen, or filter the messages. Second, you need to be able to send the messages. Let's look at sending them first.
Sending Windows Messages via the SendMessage API
Sending Windows Messages is easy. First of all you'll need to p/invoke the SendMessage Win32API. Add the DllImport to your code and you're ready to go.
[DllImport("user32.dll", CharSet=CharSet.Auto, SetLastError=true)]
private static extern IntPtr SendMessage(IntPtr hwnd, uint Msg, IntPtr wParam, IntPtr lParam);
With the DllImport added, you'll need to know a little bit about what the parameters are about. For this example, we'll just focus on the first two. The first is the handle for the application that you'll be sending the message to, the second is the message you're sending. I'll blog at a later date about how to use the wParam and lParam to send additional data with the message.
For this example we'll define our own messages to send to our applications. We could define messages that mean a number of different things to our app, but we'll just define a simple generic one that we'll send to other instances of our sample app. Choose a unique int and assign it to a const to give it some meaning. Something like this:
private const int RF_TESTMESSAGE = 0xA123;
Now for sending the message. Let's say in our sample application we want to send this message to all other running instances of our app. What we'll need to do is get all running instances of our application and send this message to each of them. An easy enough task. Take a look at the code.
//get this running process
Process proc = Process.GetCurrentProcess();
//get all other (possible) running instances
Process[] processes = Process.GetProcessesByName(proc.ProcessName);
if (processes.Length > 1)
{
//iterate through all running target applications
foreach (Process p in processes)
{
if (p.Id != proc.Id)
{
//now send the RF_TESTMESSAGE to the running instance
SendMessage(p.MainWindowHandle, RF_TESTMESSAGE, IntPtr.Zero, IntPtr.Zero);
}
}
}
else
{
MessageBox.Show("No other running applications found.");
}
That will cover sending the message to all running instances of our app (assuming we have multiple instances of the app running). Now we just need to set up the ability to receive and act on the messages.
Receiving Windows Messages by overriding the WndProc
To listen for the message we need to override the base Form class' WndProc method (Well, the WndProc is really part of the Control base class, but since the Form class is in it's downstream we'll use it there). To use the WndProc we need to override it so we can look for our specific messages. Once we do that the rest is a peice of cake. We just test for our message and do whatever action we need to perform. In this sample, we'll just indicate that we received the message by adding a line to a ListBox.
protected override void WndProc(ref Message message)
{
//filter the RF_TESTMESSAGE
if (message.Msg == RF_TESTMESSAGE)
{
//display that we recieved the message, of course we could do
//something else more important here.
this.listBox1.Items.Add("Received message RF_TESTMESSAGE");
}
//be sure to pass along all messages to the base also
base.WndProc(ref message);
}
That's it. Now you can start up multiple instances of the app and send messages from one of them to all the other running instances. You can also download a sample project that includes the code from this post. Start up multiple instances of the app and send messages to the other running instances.
Download sample project: SendMessageDemo.zip
|
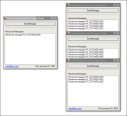 (Click to view sample) |
|